JavaScript 30 Days Challenge
本文最后更新于:2022-09-21 01:51:47 UTC+08:00
项目地址:https://github.com/ligen131/JavaScript30
教程官网:https://javascript30.com/
本文记录做 Challenge 时的一些笔记和对教程方法的部分优化与拓展。
目录
项目 | 效果演示 | 主要内容 |
---|---|---|
01 - JavaScript Drum Kit | Github Page | document.querySelector , element.addEventListener |
02 - JS and CSS Clock | Github Page | CSS 动画 transform , transition |
03 - CSS Variables | Github Page | CSS 自定义变量并修改其值 document.documentElement.style.setProperty |
04 - Array Cardio Day 1 | Github Page | JavaScript 数组相关操作 filter() , map() , sort() , reduce() |
05 - Flex Panel Gallery | Github Page | CSS flex |
06 - Type Ahead | Github Page | fetch() , 正则表达式 RegExp , CSS perspective() |
07 - Array Cardio Day 2 | Github Page | JavaScript 数组相关操作 some() , every() , find() , findIndex() , splice() , slice() , ... 扩展运算符 |
08 - Fun with HTML5 Canvas | Github Page | HTML5 canvas , CSS hsl() 等颜色函数, 鼠标事件 |
01 - JavaScript Drum Kit
发现问题:键盘按住不放会导致放开后按键(key
) classList
一直处于 "key playing"
激活状态状态卡死,如下图。
解决:将 classList.add()
改为切换。
1 |
|
拓展:增加了鼠标点击按键播放
1 |
|
02 - JS and CSS Clock
JS 中直接修改 style
会嵌入 HTML 中,具有除 !important
之外的最高优先级。
设置定时任务(Heart Beat)
1 |
|
问题:每过一分钟,秒针都会从 450deg 瞬移到 90deg,效果是逆时针快速地转了一整圈,无法和下一分钟平滑过渡。分针、时针在分别过一小时、12 小时时同理。
解决:可以用变量记录已经转过的度数,每秒对应指针加上相应的度数即可,效果是跨过 0 秒时会从 450deg 变成 456deg,可以平滑过渡。或者,对 0 秒进行特判,使指针 style.transitionDuration = "0s"
。
第一种方案实现:
1 |
|
03 - CSS Variables
CSS 图片模糊
1 |
|
问题:input.addEventListener
的事件若使用教程中的 change
会导致调色板需要选色结束退出后才生效(部分浏览器),使用 input
可以实时生效。
1 |
|
一开始用之前的 addEventListener
和 style
手动更改。
1 |
|
04 - Array Cardio Day 1
ES6 直接返回函数语法 (value) => value * value
。
Array.prototype.sort(compareFunc)
比较函数有两个参数 a, b
,需要交换(a 在 b 后)返回大于 0 的值,不需交换(a 在 b 前)返回小于 0 的值,保持原样(依浏览器而定,有些 a 在 b 前,有些 a 在 b 后)返回 0。一般而言,需要交换返回 1,无需交换返回 -1。如下列升序排序数组
1 |
|
JS 中 const
数组经 arr.sort()
排序后仍然改变其值的顺序。🤔
05 - Flex Panel Gallery
贴几个 flex 相关链接
- 教程里提到的 https://flexbox.io/
- 图示很好 https://medium.com/@js_tut/complete-flex-tutorial-69b87e5fa947 翻译 https://blog.csdn.net/weixin_26712121/article/details/108925005
- https://cloud.tencent.com/developer/article/1354252
- Mozilla 官方文档 https://developer.mozilla.org/zh-CN/docs/Web/CSS/flex
- CSS 规范 https://www.shejidaren.com/css-written-specifications.html 这个网站还有很多 CSS 相关的好玩的东西 https://www.shejidaren.com/button-hover-and-click-effects.html
第一次知道 CSS 还有这么复杂的东西,看起来 CSS 引擎也是一个巨大的工程。
惯例先把图片存到本地,云端加载太慢。
问题:过快连续点击两次(双击)会导致无法触发 transitionend
事件,导致 panel.classList.toggle('active')
在 panel
处于 open
状态时仍然触发上下文字的移动,效果如下图。(左图:均未处于 open
状态,但左边 LET'S 被双击导致错误地处于 active
状态;右图:均处于 open
状态,但左边 LET'S 被双击导致错误地处于非 active
状态)
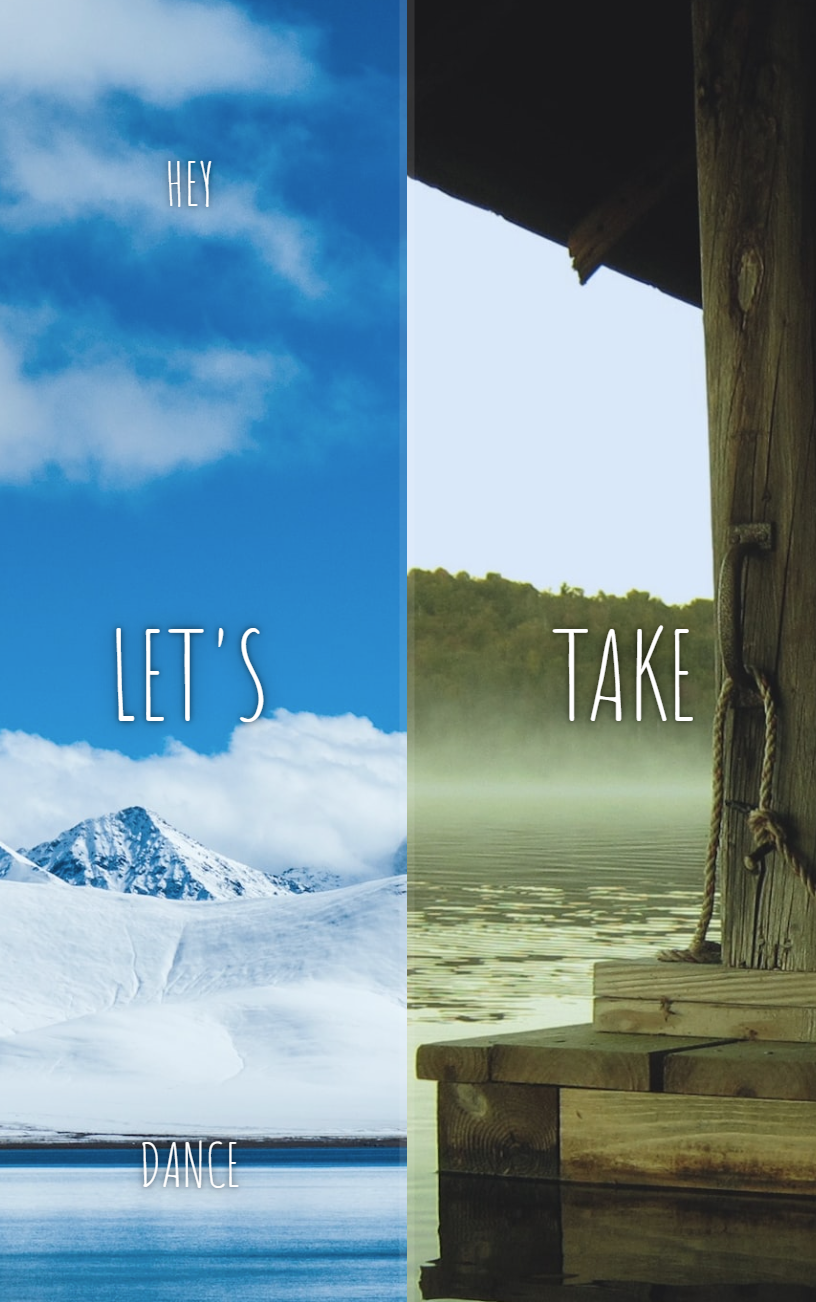
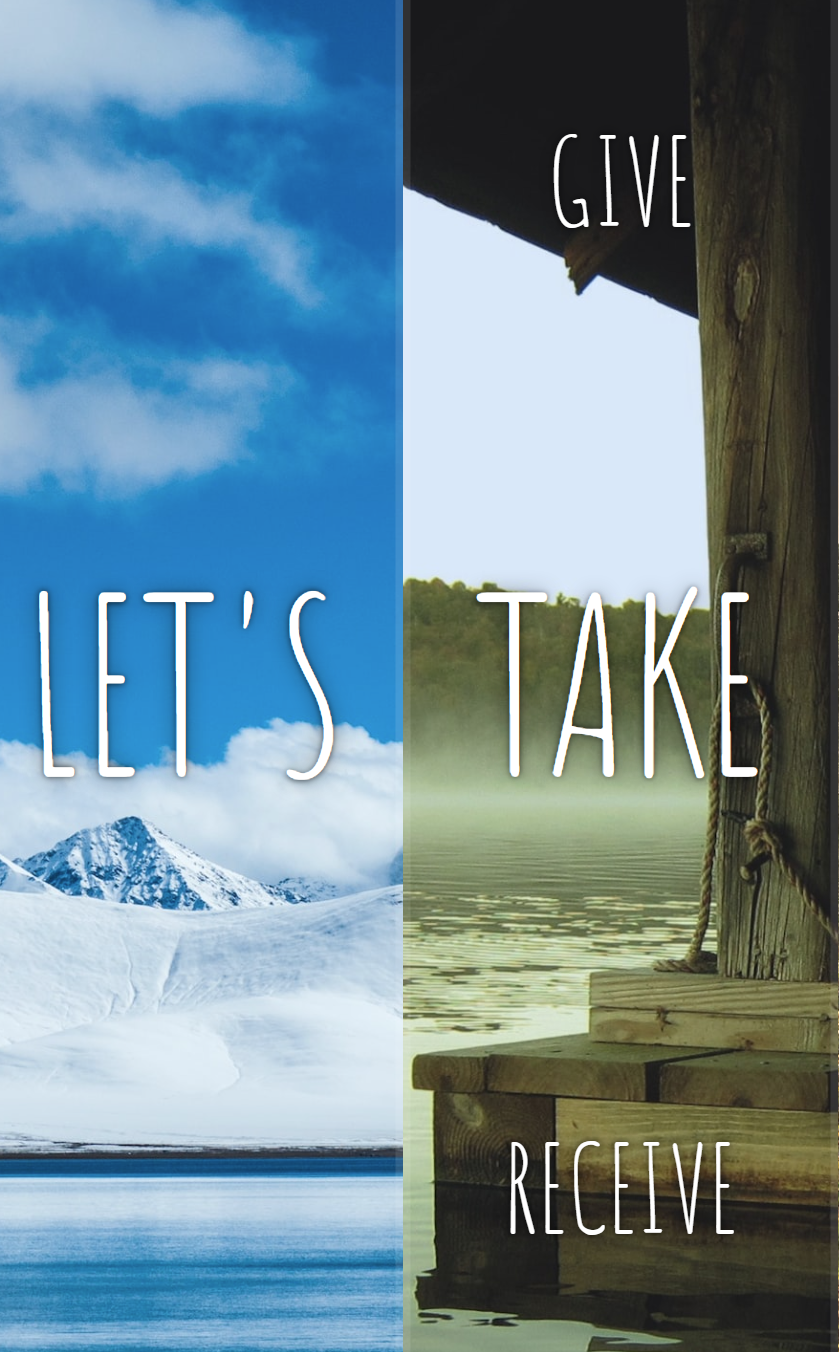
解决:分是否处于 open
状态结束的 transitionend
事件两种情况讨论,当触发 transitionend
事件时判断 panel.classList
是否存在 open
类(下面实现用 querySelector()
判断是否存在处于 open
状态的 panel
)。
1 |
|
06 - Type Ahead
表格 CSS 样式用到了 perspactive
透视,值越大代表离 z 平面越远。参考:https://css-tricks.com/almanac/properties/p/perspective/;Mozilla 官方文档:https://developer.mozilla.org/en-US/docs/Web/CSS/transform-function/perspective。
由于涉及到大小写模糊查找的问题,includes()
, match()
, replace(string, string)
都区分大小写,故只能用正则表达式解决。modifiers
包含 "i"
可以使其不区分大小写。
1 |
|
07 - Array Cardio Day 2
对象中的扩展运算符 ...
用于取出参数对象中的所有可遍历属性,拷贝到当前对象之中。
1 |
|
08 - Fun with HTML5 Canvas
HTML5 Canvas 教程:https://blog.csdn.net/u012468376/article/details/73350998
CSS 常用颜色函数:https://cloud.tencent.com/developer/article/1849536
CSS hsl()
:https://blog.csdn.net/liuhao9999/article/details/125973912